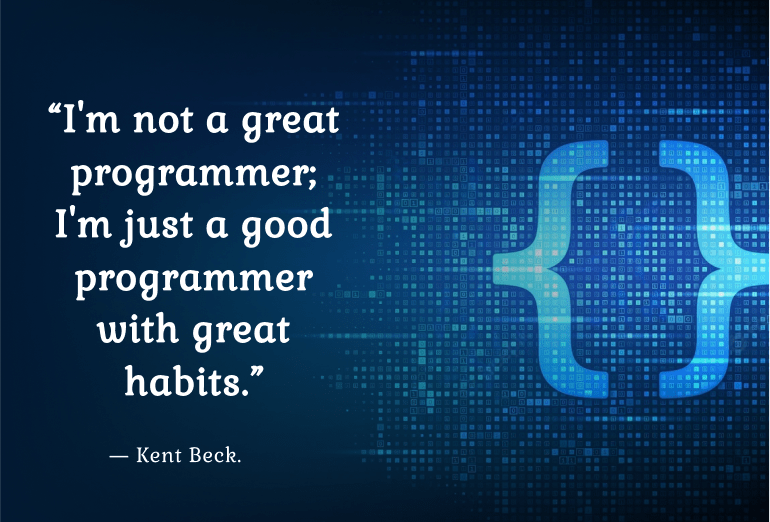
Introduction
Why write five lines of code when one will do? In programming, efficiency matters—not just in execution but in writing clean, concise, and readable code. One-liners can often replace multi-step operations, making your scripts faster, more elegant, and easier to maintain. In this post, we’ll explore Python, JavaScript, and Shell scripting tricks that can save you time and effort.
🔥 Python One-Liners
1. Instantly Swap Two Variables
Instead of the traditional method:
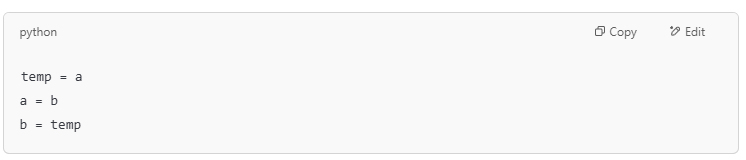
Use Python’s tuple unpacking:

This elegant swap eliminates the need for a temporary variable.
2. Reverse Any String Effortlessly

Using slicing ([::-1]
) is a neat trick to reverse strings and lists in one go.
3. Find the Most Common Element in a List

Need to quickly determine the most frequent item? The Counter
module does it in just one line.
🔥 JavaScript One-Liners
4. Convert a NodeList to an Array in Seconds

This converts NodeLists into arrays, enabling you to use array methods like .map()
and .filter()
easily.
5. Generate a Random Hexadecimal Color
Run this and get a random hex color—perfect for quick UI prototyping.

6. Check if an Array Includes a Value
No need for indexOf(), just use .includes() to verify if a value exists in an array.

🔥 Shell Scripting One-Liners
7. Remove All .log
Files in a Directory (Recursively)
Need to clear out log files? This command deletes them from the current folder and all subdirectories.

8. Start a Local Web Server Instantly
Run this, and your current directory is now a local server accessible via http://localhost:8000.

9. Replace a Word Across Multiple Files in a Directory
If you need to update a term across several files, this one-liner gets the job done efficiently.

Conclusion
Mastering one-liners is a game-changer for developers. These shortcuts improve workflow, reduce complexity, and enhance code readability. Try them out in your projects, and share which one you found most useful!
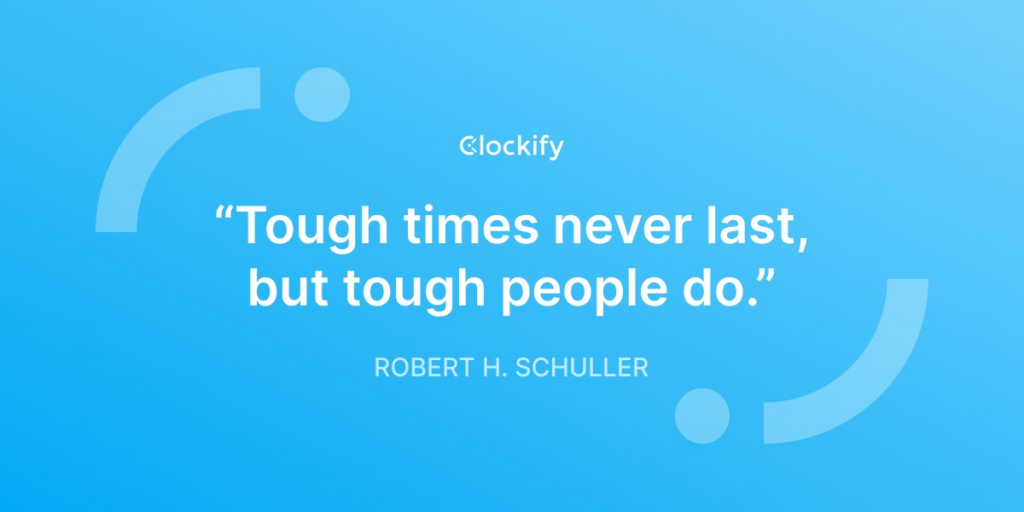